War Game Simulator
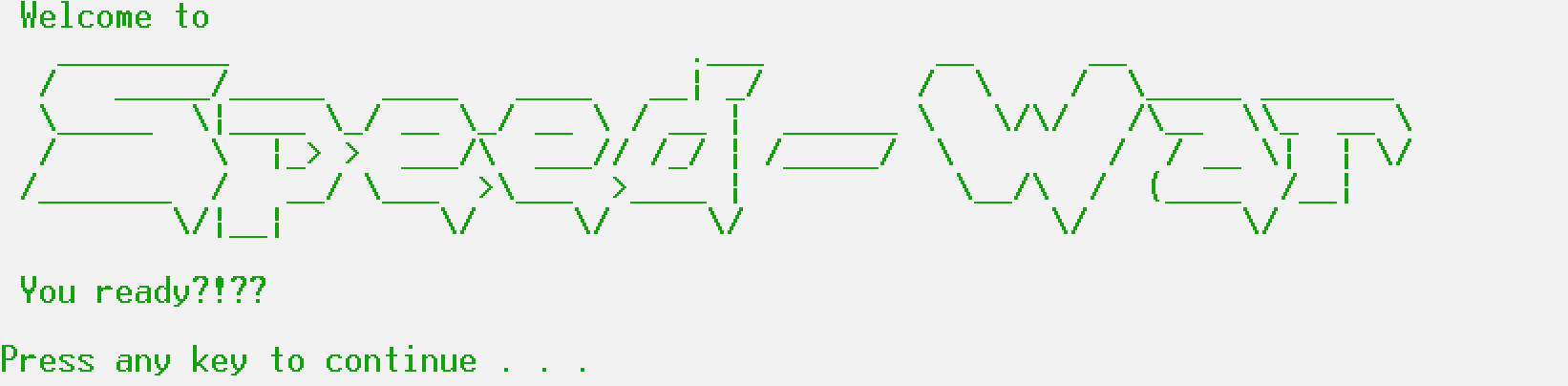
A C++ program that simulates the 2 player card game War. The 2 players are given 26 cards each from a standard card deck consisting of 52 cards. Players can take or lose cards dependent on the number of their opponents card. The game continues until a player has no cards remaining and a winner is declared as the player with all 52 cards.
How Does It Work?Using structures, a card object is represented by its rank (ranging from 2-14) and its corresponding suit (club, diamond, heart, spade). Similarly, a structure is used to create a deck object. A deck is defined by an array of cards and the number of cards in the deck.
Creating a Deck
A deck is made by utilizing a for loop iterating in consecutive increasing intervals from 2 to 14.
Within the for loop is yet another for loop iterating between values of 1 to 4, where each value
from 1-4 represents a suit. Dependent on the suit, an if statement will call upon the Card constructor and create a new card.
The card is then saved to the card array in the deck object.
Shuffling a Deck
A deck is shuffled by calling a shuffle function. Within the function, a for loop iterates 52 times (starting from 0 and ending
at 51), and a random card index is generated from 0-51 each time. A card from the deck at the randomly chosen index is then saved to a
temporary card. The the card within the deck corresponding to the for loops iteration value will turn into the randomly chosen card
from the deck (the card chosen by the random number between 0-51). Finally, the card at the randomly chosen index is replaced with the
temporary card that was earlier saved.
A card is taken from the top of a players deck by calling a function that has the deck to remove the card from, and the index of the card in the deck as its parameters. The card at the given index is removed and the card count of the deck decreases by 1.
Inserting a Card to a Deck
A card is inserted to a deck through a function that takes the deck to insert the card to, the card index, and the card to insert as its parameters. The function will move all cards in the deck beginning from the index to the total number of cards one index up in a for loop. Once all cards have been moved up in the deck, the card to be inserted is placed at the card index in the deck.
Dealing a Deck
As War is a 2 player game, both players are made a deck object and cards are distributed from the shuffled deck containnig all 52 cards. The cards are dealt using a for loop iterating 52 times. Each iteration of an odd number will give player 1 a card and an odd iteration will give player 2 a card from the deck. This is done by calling the insertCard function for the players decks and the takeCard function for the original deck with 52 cards.
Skills Applied
To view the code and the .exe file: